java数据类型:集合存储元素类型限制<泛型> ;自定义泛型<T>;派生子类泛型<T> super(泛型内参数)
时间:2021-04-12 12:33:27
收藏:0
阅读:0
问题
Java 集合有个缺点,把一个对象"丢进"集合里之后,集合就会"忘记"这个对象的数据类型,当再次取出该对象时 该对象的编译类型就变Object类型(其运行时类型没变),Java集合之所以被设计成这样,是因为集合的设计者不知道我们会用集合来保存什么类型的对象所以他们把集合设计成能保存任何类型的对象,只要求具有很好的通用性。但是,有可能使用过程中不符合预期,导致运行时报错。
import java.util.ArrayList; import java.util.List; public class ProblemTest { public static void main(String[] args) { List persons = new ArrayList(); persons.add("李一桐"); persons.add("刘亦菲"); persons.add("鞠婧祎"); //不小心加入了一个int类型的数据 persons.add(10); persons.forEach(person -> System.out.println(((String) person).length())); } }
泛型指定集合存储元素的类型
语法:
- 集合类<元素类型..> = new 集合类<>;
Set<String> persons = new HashSet<>(); Map<String, Integer> persons = new HashMap<>();
自定义泛型
我们如何自定义泛型?
Iterator对象定义泛型:
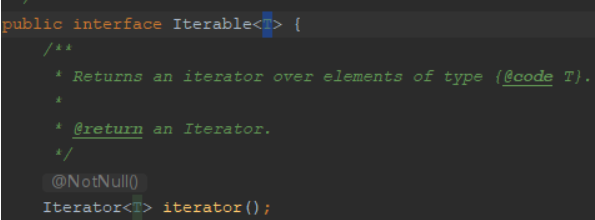
Map定义泛型:
我们就是在类名的后面加上类型的替代符,比如上面的T,K,V。我们在使用的时候,就直接把这些T,K,V替换成对应的类型名称就好了。
/** * @ClassName MyDefineClassInitTypeExample * @projectName: object1 * @author: Zhangmingda * @description: 自定义泛型测试 * date: 2021/4/11. */ public class MyDefineClassInitTypeExample { private static class Student<T>{ private T type; public Student(T type) { this.type = type; } public T getType() { return type; } } public static void main(String[] args) { Student<String> student = new Student<>("高中"); System.out.println(student.getType());//高中 Student<Integer> student1 = new Student<>(202101); System.out.println(student1.getType());//202101 } }
从泛型派生子类
当创建了带泛型申明的接口或父类之后,可以为该接口创建实现类或创建该类派生子类。子类必须指定父类的泛型具体类型,或者子类必须实现和父类相同的泛型
比如下面代码是不行的:
private static class ElementaryStudent extends Student<T>{ public ElementaryStudent(T type){ super(type); } }
但是我们可以指定具体父类泛型派生一个子类:
public class MyDefineClassInitTypeExample { private static class Student<T>{ private T type; public Student(T type) { this.type = type; } public T getType() { return type; } } private static class ElementaryStudent extends Student<String>{ public ElementaryStudent(String type){ super(type); } } public static void main(String[] args) { ElementaryStudent elementaryStudent = new ElementaryStudent("小学"); System.out.println(elementaryStudent.getType()); //小学 } }
定义一个子类的时候,无法指定父类泛型的具体类型:则
/** * @ClassName MyDefineClassInitTypeExample * @projectName: object1 * @author: Zhangmingda * @description: 自定义泛型测试 * date: 2021/4/11. */ public class MyDefineClassInitTypeExample { private static class Student<T>{ private T type; public Student(T type) { this.type = type; } public T getType() { return type; } } private static class ElementaryStudent<T> extends Student<T>{ public ElementaryStudent(T type){ super(type); } } public static void main(String[] args) { ElementaryStudent<Integer> elementaryStudent1 = new ElementaryStudent<>(202107); System.out.println(elementaryStudent1.getType()); } }
不存在泛型类:
- 用一个支持泛型的类,创建不同类型的实例,他们还是同一个类的实现。
ElementaryStudent<Integer> elementaryStudent1 = new ElementaryStudent<>(202107); System.out.println(elementaryStudent1.getType()); ElementaryStudent<String> elementaryStudent2 = new ElementaryStudent<>("幼儿园"); System.out.println(elementaryStudent2.getType()); System.out.println(elementaryStudent1.getClass()); //class MyDefineClassInitTypeExample$ElementaryStudent System.out.println(elementaryStudent2.getClass()); //class MyDefineClassInitTypeExample$ElementaryStudent System.out.println(elementaryStudent.getClass().equals(elementaryStudent2.getClass()));//true
评论(0)